ChannelWindow
Overview
ChannelWindow
stores a sequence of MaxOne/Two data frames so they can be
all be processed together, as opposed to examing individual frames. A
ChannelWindow
of length N
is initialized with the first N
frames of
the experiment.
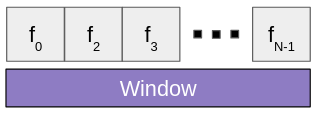
Useful chip activity data can be extracted from the window, such as the firing rate and the average x-position of the spikes. For each new frame added to the window, the oldest frame is removed.
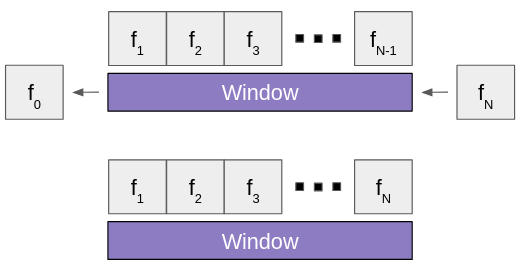
If specified, the ChannelWindow
will save the data for P
number of past
windows. Data is saved for each unique window, which means that none of the
frames in the current window are in the most recently saved window.
For example, if the window is 200 frames long, then frames 0 through 199 are saved as the first past window. The next window that will be saved will contain frames 200 through 299, since that is the first set of frames where there is no overlap with the most recently saved window.
Similar to how ChannelWindow
handles frames, when there are P
past
windows and a new window is saved, the oldest window will be removed.
Currently, the only window data that is saved is the firing rate, but this can easily be extended.
Example:
Step-by-Step
This example assumes that P
= 2, meaning that up to 2 past windows will
be saved. The first instance of the window is unique, so its firing rate is
saved.
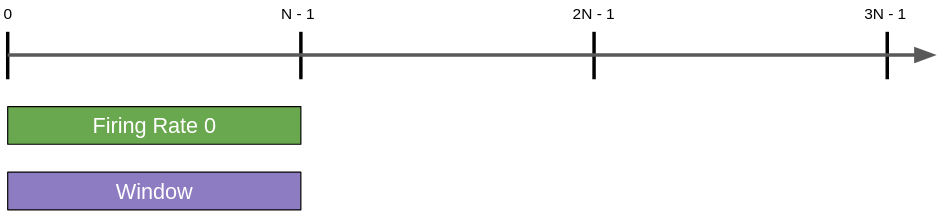
The window progresses.
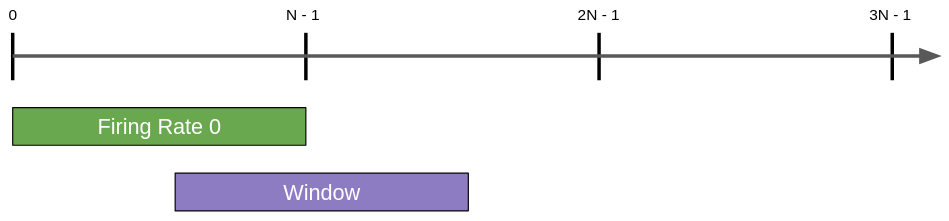
As soon as the window does not overlap with the most recently saved window, its firing rate is saved.
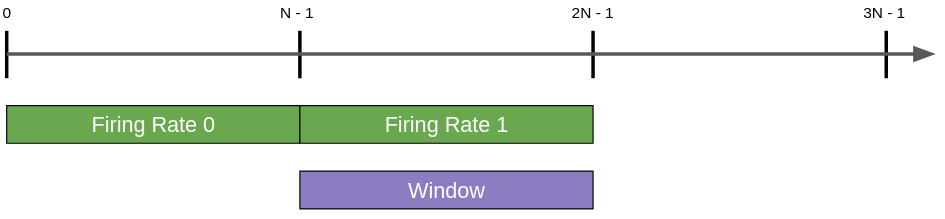
The window progresses.
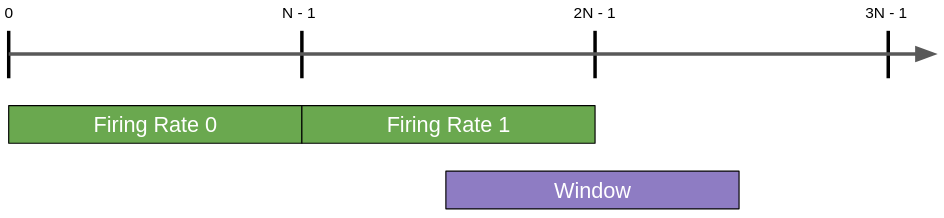
If there are P
(2 in this case) past windows, then the oldest one is
removed whenever a new one is saved.
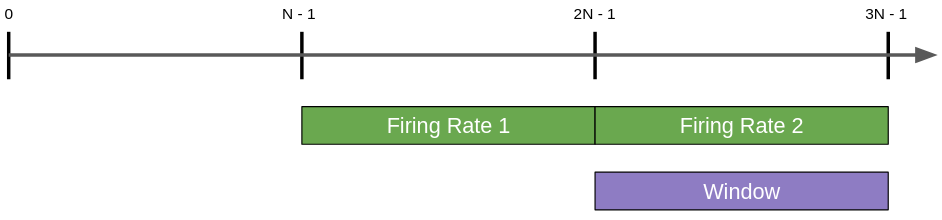
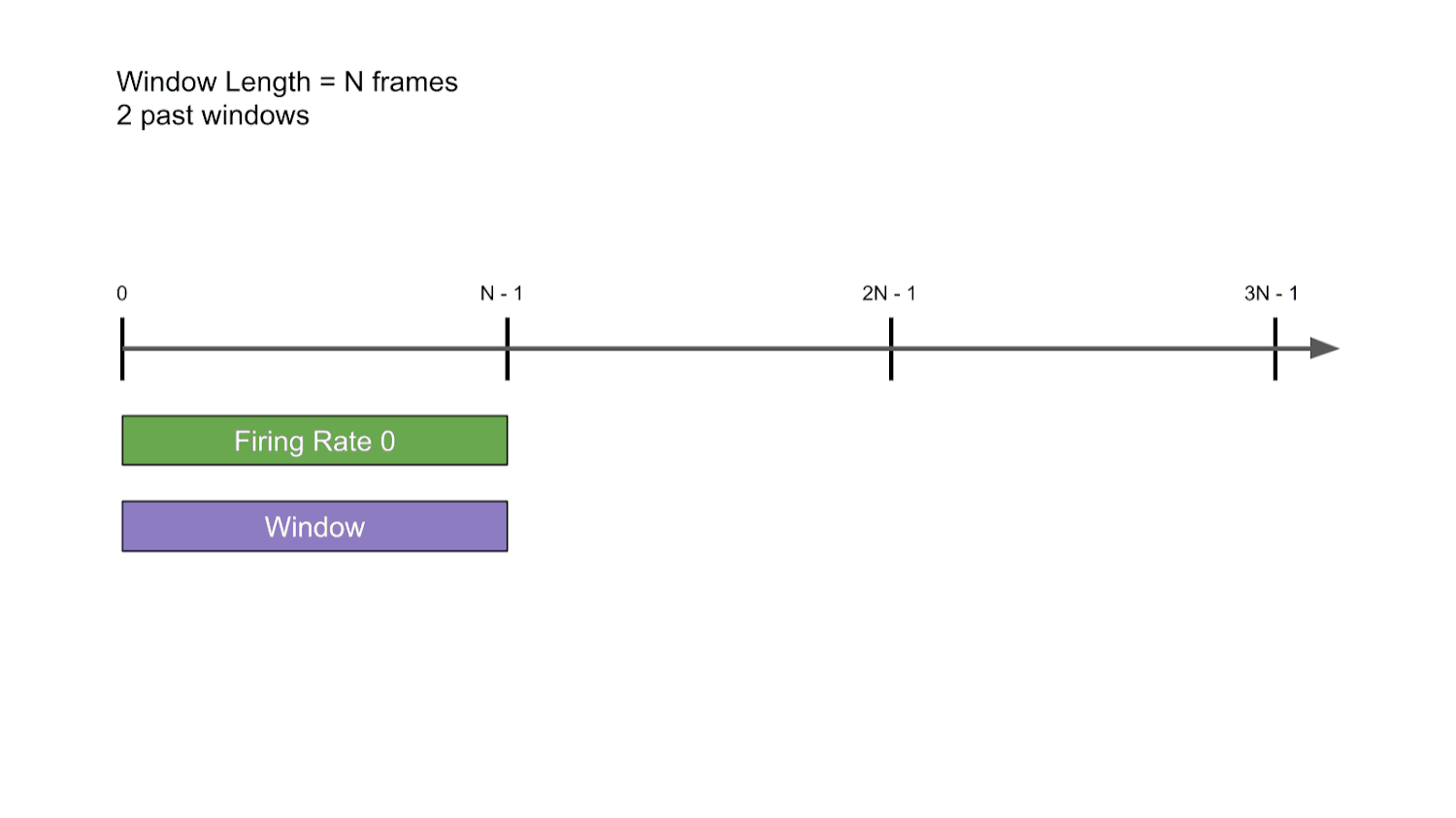
ChannelWindow
uses ElectrodeMap
to filter out any unwanted frames; this
behaviour is particularly helpful if there are multiple windows in an experiment,
where each window represents a different part of the chip.
Members
-
class ChannelWindow
Add description for the
ChannelWindow
classPublic Functions
-
ChannelWindow(int length, const char *configPath, int toSave = 0)
Constructor.
Creates a new ChannelWindow object
- Parameters:
length –
int
representing the length of the window (measured in frames)configPath –
char
array representing the full path of the config to use for the underlying ElectrodeMaptoSave –
int
indicating how many past window firing rates are to be saved, default=0
-
ChannelWindow(int length, ElectrodeMap emap, int toSave = 0)
Constructor.
Creates a new ChannelWindow object
- Parameters:
length –
int
representing the length of the window (measured in frames)emap –
ElectrodeMap
holding the current electrode config filetoSave –
int
indicating how many past window firing rates are to be saved, default=0
-
void init()
Initialize the window.
This will read in
maxlab::FilteredFrameData
structs until the window is full. The data streamer must be initialized before calling this function.
-
void shift(maxlab::FilteredFrameData frame)
Shifts window, appending new data frame to the end.
Shifts the window by removing the first frame, updating the total sum accordingly, and adding the new frame to the end. Similar to add, the sum will only be incremented for each channel in frame that exists in the electrode map. Note that it will only count the
maxlab::spikeEvents
that are found in the given config.If the window has no been initialized, this function will simply add the frame to the end of the window.
- Parameters:
frame –
maxlab::FilteredFrameData
struct to add to the window
-
maxlab::FilteredFrameData get(int idx)
Gets a
maxlab::FilteredFrameData
struct at the specified index.- Parameters:
idx –
int
representing the index of the target frame- Returns:
maxlab::FilteredFrameData
struct at the specified index within the window
-
int length()
Returns the length of the window.
- Returns:
int
-
int totalSpikeCount()
Returns the sum of all the spike counts (of valid channels) in the window.
- Returns:
int
-
int numChannels()
Returns the number of channels that the window tracks.
- Returns:
int
-
ElectrodeMap electrodeMap()
Returns the
ElectrodeMap
- Returns:
ElectrodeMap
containing all of the channel, electrode mappings
-
float avgPastWindows()
Gets a vectors containing the firing rates of all the past windows.
Returns the average firing rate of all the past windows
- Returns:
vector<float>
- Returns:
float
-
float getAvgX()
Computes the average x coordinate of all the spike events in the window, counting each channel only once.
- Returns:
float
-
float firingRateSD()
Computes the standard deviation of the firing rates of all past windows.
- Returns:
float
-
float firingRate()
Computes the firing rate of the window.
- Returns:
float
-
bool pastWindowsReady()
Indicates whether the window is completely unique from the past windows.
The past windows are stored end-to-end, so, at any point in time, there is a high chance that the current window overlaps with the past ones. When this function returns true, the current window does not overlap with any of the previous windows, and will be counted in all computations relating to the past windows.
- Returns:
bool
-
void print()
Prints the ChannelWindow.
-
void debug(string message)
Prints the window with a message.
Also counts each spike event in the window manually, and compares it to the recorded sum
- Parameters:
message –
string
to print
-
ChannelWindow(int length, const char *configPath, int toSave = 0)